Content - Guide
Create and manage your game's live content [PROF-Content-02]
The purpose of this feature is to allow game maker to store project-specific data objects. In this guide, we will create and publish a content entry, then pull the content from the server within an instance of Unity's MonoBehaviour
script.
Adding Content
Game makers may create new content of existing content types or new content of custom content types. For the purposes of this guide, we will be using the existing "Item" type.
Step | Detail |
---|---|
1. Open the Content Manager Window | • Unity → Window → Beamable → Open Content Manager |
2. Create the "Item" content | ![]() • Select the content type in the list • Press the "Create" button • Populate the content name |
3. Open the Unity Project Window | • Unity → Window → General→ Project |
4. Select the "Item" asset | • Search by the name given in step #3 • View the asset in the Unity Inspector Window |
5. Populate all data fields | ![]() Note: The icons can be set to any valid Unity’s Addressable object. |
6. Save the Unity Project | • Unity → File → Save Project Best Practice: If you are working on a team, commit to version control in this step. |
7. Publish the content | • Press the "Publish" button in the Content Manager Window |
Downloading Content
Content that has been published to the server can be pulled from Beamable's ContentService via a ContentLink or ContentRef. Both are shown in this example.
using System;
using Beamable.Common.Content;
using Beamable.Common.Inventory;
using UnityEngine;
namespace Beamable.Examples.Services.ContentService
{
[Serializable]
public class ItemLink : ContentLink<ItemContent> {}
/// <summary>
/// Demonstrates <see cref="ContentService"/>.
/// </summary>
public class ContentServiceExistingExample : MonoBehaviour
{
// Fields ---------------------------------------
[SerializeField] private ItemLink _itemLink;
[SerializeField] private ItemRef _itemRef;
private ItemContent _itemContentFromLink = null;
private ItemContent _itemContentFromRef = null;
// Unity Methods --------------------------------
protected void Start()
{
Debug.Log($"Start()");
SetupBeamable();
}
// Methods --------------------------------------
private async void SetupBeamable()
{
var beamContext = BeamContext.Default;
await beamContext.OnReady;
Debug.Log($"beamContext.PlayerId = {beamContext.PlayerId}");
await _itemLink.Resolve()
.Then(content =>
{
_itemContentFromLink = content;
Debug.Log($"_itemContentFromLink.Resolve() Success! " +
$"Id = {_itemContentFromLink.Id}");
})
.Error(ex =>
{
Debug.LogError($"_itemContentFromLink.Resolve() Error!");
});
await _itemRef.Resolve()
.Then(content =>
{
_itemContentFromRef = content;
Debug.Log($"_itemContentFromRef.Resolve() Success! " +
$"Id = {_itemContentFromRef.Id}");
}).Error(ex =>
{
Debug.LogError($"_itemContentFromRef.Resolve() Error!");
});
}
}
}
Learning Fundamentals
Game makers who are new to Unity and C# can review the fundamentals here.
The above code sample uses C# async methods. However, the same code can be written using the Promise library.• See Beamable: Asynchronous Programming for more info
Inspector
After being added to a GameObject, the Inspector will display a drop-down of all valid content entries.
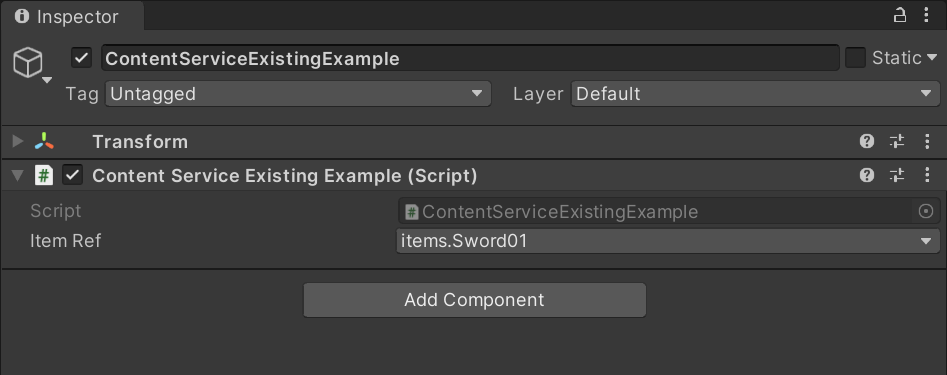
Output
Click Play in the Editor and inspect the console to see that the Item content was pulled successfully.
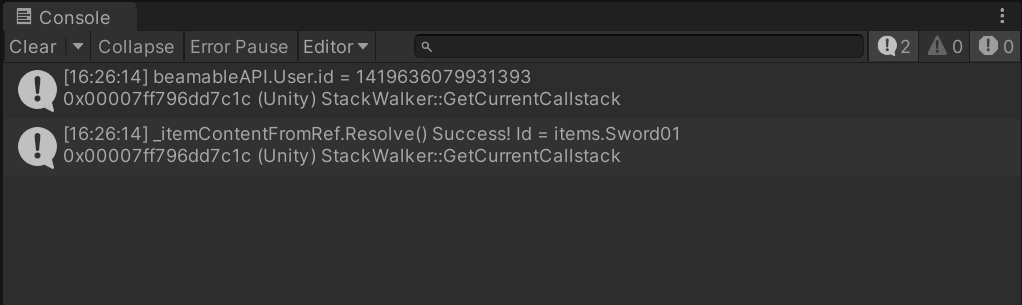
Switching Namespaces
To enable multiple namespaces, go to Project Settings→Beamable→Content and press the "Enable Multiple Content Namespaces" checkbox.
After that, a dropdown option should appear on the "Publish" button in the Content Manager header. Choose "Publish new Content namespace" to create a new namespace.
Enter a name for the new namespace, then press "Publish" to create it.
Your namespaces will now show up beside your environments in the Content Manager. The selected namespace will be the one you currently are working with, and you can easily switch between namespaces by clicking on the wanted namespace in the dropdown.
Updated over 1 year ago