Announcements - Code
[LIVE-Announcements-03]
Prerequisites
This guide assumes the following prerequisites have been completed:
Retrieving announcements via C# code is quite simple, and resembles the Content syntax. This is a more flexible solution, since game makers can fit the announcement content to their own UI, rather than using the UI packaged with the Announcements Prefab.
Step 1. Initialize BeamContext
Before we can perform any actions, the current player must be logged in and Beamable must be initialized.
private BeamContext _beamContext;
private async void Start()
{
_beamContext = BeamContext.Default;
await _beamContext.OnReady;
Debug.Log($"User Id: {_beamContext.PlayerId}");
}
Step 2a. Retrieve Announcements via GetCurrent
The simplest way to retrieve announcements is via the GetCurrent()
method from the AnnouncementService
. This will return an AnnouncementQueryResponse
, which contains a list of Announcements.
private async Task<List<AnnouncementView>> GetAnnouncements()
{
var response = await _beamContext.Api.AnnouncementService.GetCurrent();
return response?.announcements;
}
Step 2b. Retrieve Announcements via Subscription
The AnnouncementService
also features a Subscribe
method, allowing the game client to react to new announcements as they are published.
private void SubscribeToAnnouncements()
{
_beamContext.Api.AnnouncementService.Subscribe(response =>
{
PrintAnnouncements(response?.announcements);
});
}
Step 3. Display Announcements
Once we have retrieved the announcements, they can be displayed in the app. The function below simply reads the title and body of the content and logs them to the console, but the specific game implementation will require some custom solution.
private void PrintAnnouncements(List<AnnouncementView> announcements)
{
foreach (var announcement in announcements)
{
Debug.Log(announcement.title);
Debug.Log(announcement.body);
}
}
Step 4. Run & Test
Call either the SubscribeToAnnouncements()
or GetAnnouncements()
functions and inspect the Unity console to validate that your functions are working. Below is the console for a successful run.
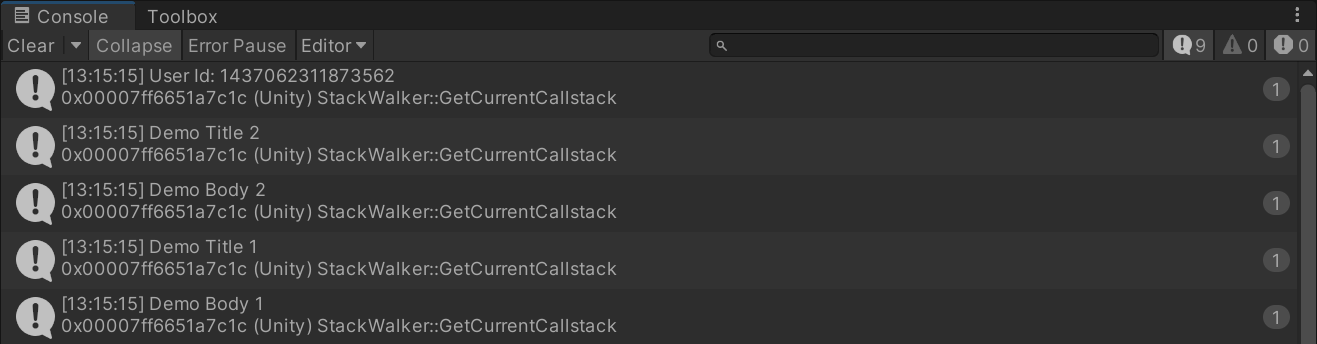
Complete Script
Below is the full script used for this guide.
using System.Collections.Generic;
using System.Threading.Tasks;
using Beamable;
using Beamable.Common.Api.Announcements;
using UnityEngine;
public class AnnouncementsTest : MonoBehaviour
{
private BeamContext _beamContext;
private async void Start()
{
_beamContext = BeamContext.Default;
await _beamContext.OnReady;
Debug.Log($"User Id: {_beamContext.PlayerId}");
var announcements = await GetAnnouncements();
PrintAnnouncements(announcements);
SubscribeToAnnouncements();
}
private async Task<List<AnnouncementView>> GetAnnouncements()
{
var response = await _beamContext.Api.AnnouncementService.GetCurrent();
return response?.announcements;
}
private void SubscribeToAnnouncements()
{
_beamContext.Api.AnnouncementService.Subscribe(response =>
{
PrintAnnouncements(response?.announcements);
});
}
private void PrintAnnouncements(List<AnnouncementView> announcements)
{
foreach (var announcement in announcements)
{
Debug.Log(announcement.title);
Debug.Log(announcement.body);
}
}
}
Updated about 1 year ago