Leaderboard (ABC) - Guide
[SMPL-ABC-02]
This document and the sample project allow game makers to understand and apply the benefits of a Leaderboard in game development. Or watch this video.
Download
These learning resources provide a better way to build live games in Unity.
Source | Detail |
---|---|
![]() | 1. Download the Leaderboard ABC Sample Project 2. Open in Unity Editor ( Version 2020.3.23f1 ) 3. Open the Beamable Toolbox 4. Sign-In / Register To Beamable. See Step 1 - Getting Started for more info 5. Open the Content Manager Window and click "Publish". See Content Manager for more info 6. Open the 1.Intro Scene7. Play The Scene: Unity → Edit → Play Note: Supports Mac & Windows and includes the Beamable SDK |
Screenshots
The player navigates from the Intro Scene to the Game Scene, where all the action takes place. The Leaderboard scene shows the high scores achieved by all players of the game.
Intro Scene | Game Scene | Leaderboard Scene | Project |
---|---|---|---|
![]() | ![]() | ![]() | ![]() |
Player Experience Flowchart
Here is the high level execution flow of user input and system interactions.
Game Maker User Experience
There are 3 major parts to this game creation process. During development, the game maker's user experience is as follows:
Steps
These steps are already complete in the sample project. The instructions here explain the process.
Follow these steps to get started:
Related Features
More details are covered in related feature page(s).
• Leaderboards - Allow player to manage leaderboard
Step 1. Setup The Project
Step | Detail |
---|---|
1. Install the Beamable SDK and Register/Login | • See Step 1 - Getting Started for more info |
Step 2. Create Content
Step | Detail |
---|---|
1. Create CurrentScore Stat Object | • See Stats - Guide for more info |
2. Create HighScore Stat Object | • See Stats - Guide for more info |
3. Open the Content Manager Window | • Unity → Window → Beamable → Open Content Manager |
4. Create the "Leaderboard" content | ![]() • Select the content type in the list • Press the "Create" button • Populate the content name |
5. Populate all data fields | ![]() NOTE: With Write_self set as true the LeaderboardService allows the game client to set the score with C#. This is required for the sample game project.* |
6. Save the Unity Project | • Unity → File → Save Project Best Practice: If you are working on a team, commit to version control in this step. |
7. Publish the content | • Press the "Publish" button in the Content Manager Window |
Step 3. Create Game Code
Name | Detail |
---|---|
1. Create C# game-specific logic | • Implement game logic • Handle player input events • Render graphics & sounds Note: This represents the bulk of the development effort. The details depend on the specifics of the game project. |
2. Play the 1.Intro Scene | • Unity → Edit → Play |
3. Enjoy the game! | • Can you beat the high score? • Checkout the Leaderboard |
4. Stop the Scene | • Unity → Edit → Stop |
Code
Here are a few highlights from the project's major classes.
In the interest of brevity, these code embeds are incomplete. Download the project to see the complete code.
The IntroSceneManager
uses Beamable's ConnectivityService
to check internet availability.
namespace Beamable.Samples.ABC
{
public class IntroSceneManager : MonoBehaviour
{
// Fields ---------------------------------------
private BeamContext _beamContext;
// Unity Methods ------------------------------
protected void Start()
{
SetupBeamable();
}
// Other Methods --------------------------------
private async void SetupBeamable()
{
_beamContext = BeamContext.Default;
await _beamContext.OnReady;
// Attempt Connection to Beamable
try
{
_isBeamableSDKInstalled = true;
// Handle any changes to the internet connectivity
_beamContext.Api.ConnectivityService.OnConnectivityChanged +=
ConnectivityService_OnConnectivityChanged;
ConnectivityService_OnConnectivityChanged
(_beamContext.Api.ConnectivityService.HasConnectivity);
if (IsDemoMode)
{
//Set my player's name
MockDataCreator.SetCurrentUserAlias(_beamContext.Api.StatsService, "This_is_you:)");
//Populate the leaderboard with at least 10 mock users/scores
PopulateLeaderboardWithMockData(_beamContext.Api.LeaderboardService);
//Set the Beamable stat(s) to have initial values
PopulateStats(_beamContext.Api.StatsService, _beamContext.Api.LeaderboardService);
}
}
catch (Exception e)
{
// Failed to connect (e.g. not logged in)
_isBeamableSDKInstalled = false;
_isBeamableSDKInstalledErrorMessage = e.Message;
ConnectivityService_OnConnectivityChanged(false);
}
}
// Event Handlers -------------------------------
private void ConnectivityService_OnConnectivityChanged(bool isConnected)
{
//React to changes in connectivity
}
// Other Code (Not Shown) ...
}
}
Inspector
Here is the GameSceneManager.cs
main entry point for the Game Scene interactivity.
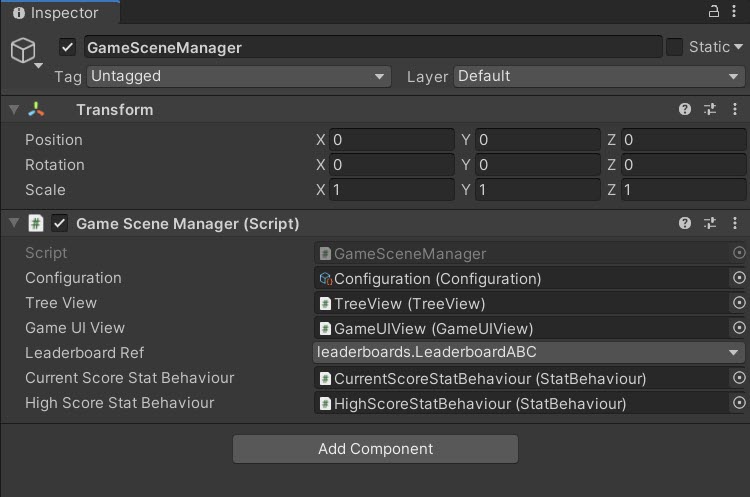
The "Leaderboard" reference is easily configurable
Here is the Configuration.cs
holding high-level, easily-configurable values used by various areas on the game code.
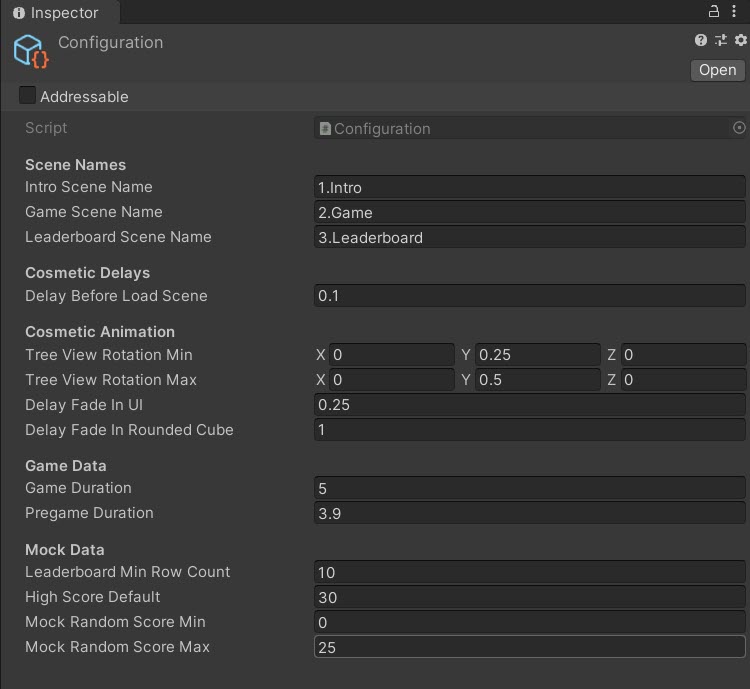
The "Configuration" values are easily configurable
Gotchas
Here are hints to help explain some of the trickier concepts:
• While the name is similar, this Configuration.cs
is wholly unrelated to Beamable's Configuration Manager.
Optional: Game Makers may experiment with new Game Duration values to allow the player's turn to occur faster or slower.
Code
The GameSceneManager
uses Beamable's StatBehaviour
to set/get player-specific stored values and uses Beamable's LeaderboardService
upon victory to submit the player-specific score.
namespace Beamable.Samples.ABC
{
public class GameSceneManager : MonoBehaviour
{
// Fields ---------------------------------------
[SerializeField]
private TreeView _treeView = null;
[SerializeField]
private StatBehaviour _currentScoreStatBehaviour = null;
private BeamContext _beamContext;
// Unity Methods ------------------------------
protected void Start ()
{
_currentScoreStatBehaviour.OnStatReceived.AddListener(CurrentScoreStatBehaviour_OnStatReceived);
SetupBeamable();
}
// Other Methods --------------------------------
private async void SetupBeamable()
{
_leaderboardContent = await _leaderboardRef.Resolve();
_beamContext = BeamContext.Default;
await _beamContext.OnReady;
try
{
RestartGame();
}
catch (Exception)
{
_gameUIView.StatusText.text = ABCHelper.InternetOfflineInstructionsText;
}
}
private void SetLeaderboardScore(LeaderboardContent leaderboardContent, double score)
{
_beamableAPI.LeaderboardService.SetScore(leaderboardContent.Id, score);
}
// Event Handlers -------------------------------
private void CurrentScoreStatBehaviour_OnStatReceived(string currentScore)
{
if (_lastGlobalHighScore == ABCConstants.UnsetValue)
{
return;
}
// Beating the high score will 'complete' the tree
float growthPercentageOf100 = (100 * Int32.Parse(value)) / _lastGlobalHighScore;
_treeView.GrowthPercentage = Mathf.Clamp01(growthPercentageOf100 / 100);
// Play sound that increases in pitch as GrowthPercentage increases
float pitch = CoreHelper.GetAudioPitchByGrowthPercentage(_treeView.GrowthPercentage);
SoundManager.Instance.PlayAudioClip(SoundConstants.Click02, pitch);
_gameUIView.StatusText.text = $"{_currentScoreStatBehaviour.Value} clicks.\n" +
$"{CoreHelper.GetRoundedTime(_gameTimeRemaining)} secs left! Keep going!";
}
// Other Code (Not Shown) ...
}
}
Additional Experiments
Here are some optional experiments game makers can complete in the sample project.
Did you complete all the experiments with success? We'd love to hear about it. Contact us.
Difficulty | Scene | Name | Detail |
---|---|---|---|
Beginner | Game | Tweak Configuration | • See Assets/ScriptableObjects/Configuration.asset .1. Change timing of game play and animations |
Beginner | Game | Add new Input | • The current game uses mouse-clicks as input for core game play. 1. Add mode where user clicks the Spacebar or other key as input |
Intermediate | Game | Add 3 Difficulty Levels | • The current game has a set difficulty and set level duration. 1. Add difficulty levels 2. Increase level duration |
Advanced | Game | Add 3 Types of Trees | • The current game has one TreeUI which grows the tree from bottom to top out of blocks and uses scale and movement as growth effects.1. Add new trees 2. Add new growth effects |
Advanced
This section contains any advanced configuration options and workflows.
Using Beamable Stats
Beamable Stats are a simple place to read/write info. (Ex: How many characters does the player own?)
See Stats for more info.
More Info
• Strictly speaking, this sample project's needs do not require Beamable Stats.
• The feature is included in this sample project for educational purposes.
Managing Leaderboard Via Portal
The Portal allows the game maker to manage Leaderboards.
See Leaderboards - Guide for more info.
Learning Resources
These learning resources provide a better way to build live games in Unity.
Source | Detail |
---|---|
![]() | 1. Download the Leaderboard ABC Sample Project 2. Open in Unity Editor ( Version 2020.3.23f1 ) 3. Open the Beamable Toolbox 4. Sign-In / Register To Beamable. See Step 1 - Getting Started for more info 5. Open the Content Manager Window and click "Publish". See Content Manager for more info 6. Open the 1.Intro Scene7. Play The Scene: Unity → Edit → Play Note: Supports Mac & Windows and includes the Beamable SDK |
Updated almost 2 years ago