Stats - Code
Store pieces of data about your players [PROF-Stats-03]
Stats does not require a specific Beamable Feature Prefab. Instead, the main entry point to this feature is C# programming.
API
Here are API highlights for StatsService
.
Method Name | Detail |
---|---|
GetStats (domain, access, type, id) | • Get one or more stat values |
SetStats (access, statsDictionary) | • Set one or more stat values |
The SetStats
and GetStats
methods require additional parameters.
Parameter Name | Detail |
---|---|
access | Possible values include “public” or “private” Public client stats can be retrieved by anyone who knows your ID. Private client stats can only be retrieved for yourself. The distinction is not meaningful in backend, but in practice “game” stats are usually also “private” |
domain | Possible values include "game" (backend) or "client" (Unity) Domain is one of “game” (backend) or “client” (Unity). Game stats can only be retrieved from microservices, but client stats can be retrieved both in microservices and in Unity code |
id | The numeric user ID of the player who owns the stats Note: For client private stats this must match the ID of your login (e.g. beamContext.PlayerId ) |
type | Possible values include only “player” Note: This parameter exists for legacy purposes only |
Steps
Follow these steps to get started:
1. Create Stats
There are no explicit steps to create a new Stat. Instead, simply write to a stat via C# and if the stat does not already exist, it will be created on-the-fly.
2. Use Stats
Write Stats
This writes to the stats of the current (logged in) player.
var beamContext = BeamContext.Default;
await beamContext.OnReady;
string access = "public";
string type = "player";
Dictionary<string, string> statsDictionary =
new Dictionary<string, string>() { { "MyExampleStat", "99" } };
await beamContext.Api.StatsService.SetStats(access, statsDictionary);
Read Stats (Public Access)
This reads from the public stats of _any _player.
var beamContext = BeamContext.Default;
await beamContext.OnReady;
long id = beamContext.PlayerId;
string access = "public";
string domain = "client";
string type = "player";
Dictionary<string, string> statsDictionary =
await beamContext.Api.StatsService.GetStats(domain, access, type, id);
Read Stats (Private Access)
For reasons of security, reading the _private _stats of any player is possible only with Beamable Microservices.
See Microservices for more info.
3. View Stats
The Portal allows the game maker to view and edit player Stats.
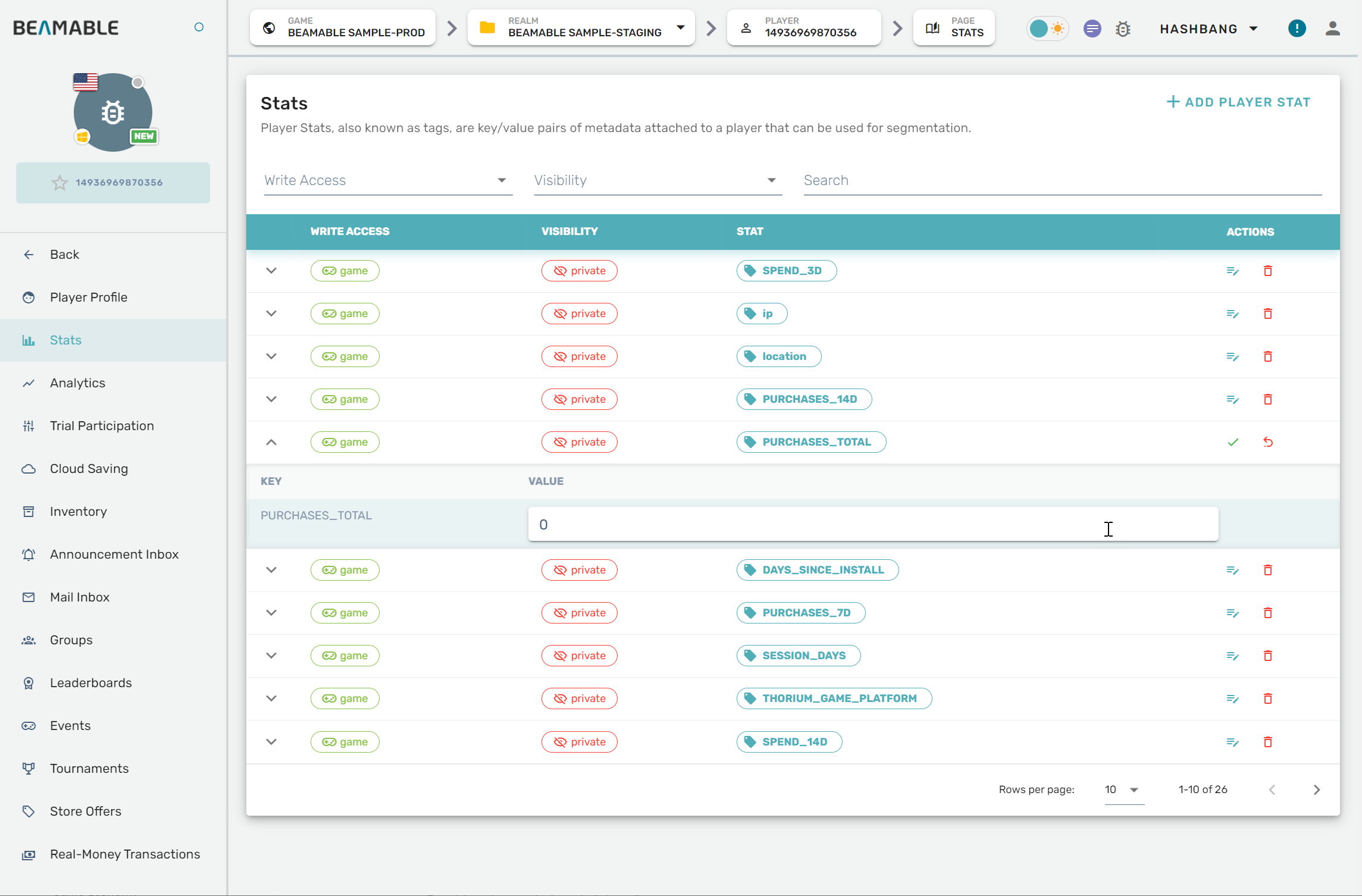
The Beamable "Portal"
Examples
Beamable SDK Examples
• The following example code is available for download at GitHub.com/Beamable_SDK_Examples
Stat Coding
In this StatCodingExample.cs
example, the C# API is used to read and write to the Stat.
This low-level solution offers high flexibility.
Inspector
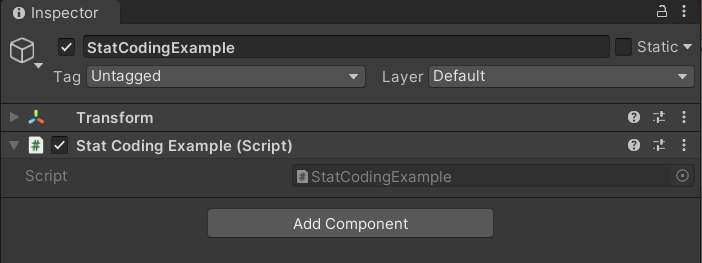
Code
using System.Collections.Generic;
using UnityEngine;
namespace Beamable.Examples.Services.StatsService
{
/// <summary>
/// Demonstrates <see cref="StatsService"/>.
/// </summary>
public class StatCodingExample : MonoBehaviour
{
// Unity Methods --------------------------------
protected void Start()
{
Debug.Log($"Start()");
SetupBeamable();
}
// Other Methods ------------------------------
private async void SetupBeamable()
{
var context = BeamContext.Default;
await context.OnReady;
Debug.Log($"context.PlayerId = {context.PlayerId}");
string statKey = "MyExampleStat";
string access = "public";
string domain = "client";
string type = "player";
long id = context.PlayerId;
// Set Value
Dictionary<string, string> setStats =
new Dictionary<string, string>() { { statKey, "99" } };
await context.Api.StatsService.SetStats(access, setStats);
// Get Value
Dictionary<string, string> getStats =
await context.Api.StatsService.GetStats(domain, access, type, id);
string myExampleStatValue = "";
getStats.TryGetValue(statKey, out myExampleStatValue);
Debug.Log($"myExampleStatValue = {myExampleStatValue}");
}
}
}
Updated about 1 year ago