Leaderboards - Guide
Allow player to manage leaderboard [SOCL-Leaderboards-02]
Variations On A Theme
Choose the Leaderboard setup that works best for your needs;
• Leaderboard (Standard) - Shows non-animated score values. See Leaderboards - Guide for more info
• Leaderboard (With Interpolation) - Shows animated score values. See Leaderboards - Code for more info
The purpose of this guide is to allow the player to see the Leaderboard.
Steps
Follow these steps to get started:
Step 1. Create Leaderboard Content
The Leaderboard content is required to properly populate the Leaderboard UI.
Step | Detail |
---|---|
1. Open the Content Manager Window | • Unity → Window → Beamable → Open Content Manager |
2. Create the "Leaderboard " content | ![]() • Select the content type in the list • Press the "Create" button • Populate the content name |
3. Open the Unity Project Window | • Unity → Window → General→ Project |
4. Select the leaderboard asset | • Search by the name given in step #3 • View the asset in the Unity Inspector Window |
5. (Optional) Configure data fields | ![]() Note: The Write_self variable allows for control of Leaderboard permissions. See the Beamable Security & Permissions callout for more info. |
6. Save the Unity Project | • Unity → File → Save Project Best Practice: If you are working on a team, commit to version control in this step. |
7. Publish the content | • Press the "Publish" button in the Content Manager Window |
Beamable Security & Permissions
Each
Leaderboard
content object asset includes awrite_self
field.• True: Allows for client-authoritative score updates. Benefits include ease-of-use for game makers and is ideal for a game which can tolerate the occasional cheater. It is hackable.
• False: Allows for server-authoritative score updates and requires additional setup via Microservices. Benefits include higher system security and is ideal for a game which requires that. It is less hackable.
Step 2. Add Leaderboard Flow Prefab
Step | Detail |
---|---|
1. Open the "Toolbox" Window | • Unity → Window → Beamable → Open Beamable Toolbox |
2. Add the "Leaderboard Flow" Prefab | • Drag this Prefab from the Beamable Toolbox Window to the Unity Hierarchy Window |
Here is the "Beamable" menu as seen in Unity:
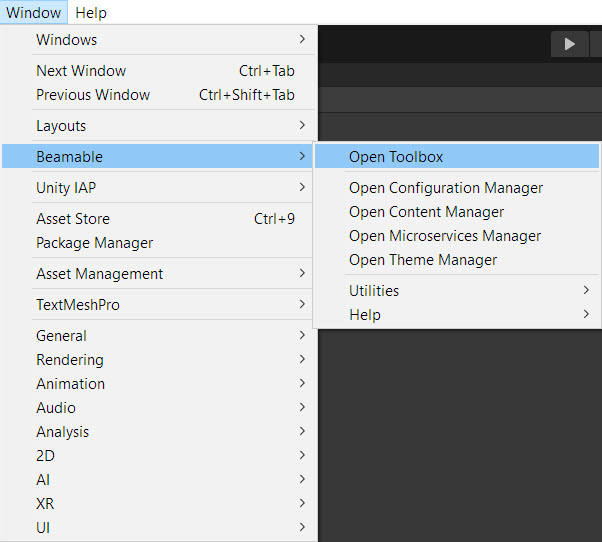
The “Beamable” Menu
Here is the feature Prefab as seen in the Beamable Toolbox.
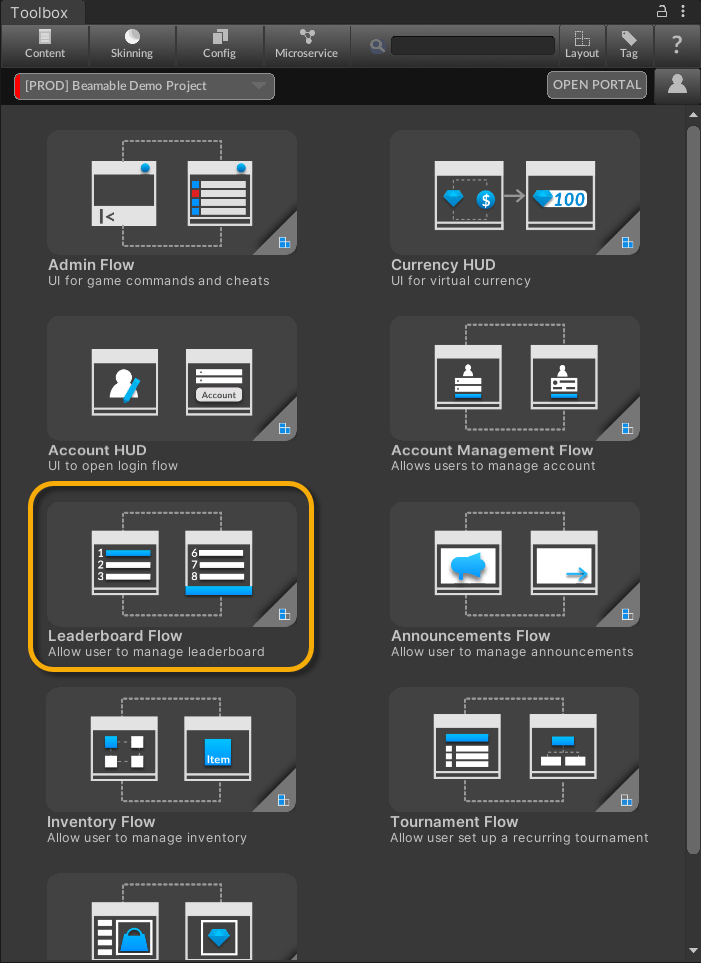
The Beamable "Leaderboard Flow" in the Beamable Toolbox Window
Step 3. Reward Score via Leaderboard
To appear on the Leaderboard, a player must have a competitive score.
During Development
The Portal allows the game maker to add/view/edit a player's score. This is especially helpful during development.
See Leaderboards - Guide » Managing Leaderboard Via Portal for more info.
During Production
To properly reward the players with score in production code, use custom C#. See the following example for inspiration.
Code
Beamable SDK Examples
• The following example code is available for download at GitHub.com/Beamable_SDK_Examples
using Beamable.Common.Leaderboards;
using UnityEngine;
namespace Beamable.Examples.Services.LeaderboardService
{
/// <summary>
/// Demonstrates <see cref="LeaderboardService"/>.
/// </summary>
public class LeaderboardServiceExample : MonoBehaviour
{
// Fields ---------------------------------------
[SerializeField] private LeaderboardRef _leaderboardRef = null;
[SerializeField] private double _score = 100;
// Unity Methods --------------------------------
protected void Start()
{
Debug.Log($"Start()");
LeaderboardServiceSetScore(_leaderboardRef.Id, _score);
}
// Methods --------------------------------------
private async void LeaderboardServiceSetScore(string id, double score)
{
var beamContext = BeamContext.Default;
await beamContext.OnReady;
Debug.Log($"beamContext.PlayerId = {beamContext.PlayerId}");
await beamContext.Api.LeaderboardService.SetScore(id, score);
Debug.Log($"LeaderboardService.SetScore({id},{score})");
}
}
}
Advanced
This section contains any advanced configuration options and workflows.
Managing Leaderboards Via Portal
The Portal allows the game maker to manage Leaderboards.
Step | Detail |
---|---|
1. Open the Portal Window 2. Select your Realm and navigate to the "Leaderboards" section | • See Portal for more info |
3. Manage all of a Realm's Leaderboards 4. Click the verticle ellipses to administer a single Leaderboard | ![]() |
5. View the Leaderboard to manage its listings | ![]() Available Operations • Delete a single score • Delete the Leaderboard • Clear the Leaderboard Note: Some of these operations cannot be undone. |
Updated 9 months ago